# 模板字符串
模板字符串是ES6(ECMAScript 2015)引入的一个新特性,它提供了一种更灵活、更直观的方式来创建字符串。以下是模板字符串的主要特点和用法:
# 1. 基本语法
模板字符串使用反引号(`)来定义,而不是单引号或双引号。
const greeting = `Hello, World!`;
console.log(greeting); // 输出: Hello, World!
# 2. 多行字符串
模板字符串可以轻松创建多行字符串,无需使用换行符(\n)。
const multiLine = `
This is a
multi-line
string.
`;
console.log(multiLine);
// 输出:
// This is a
// multi-line
// string.
# 3. 插值表达式
模板字符串允许在字符串中嵌入表达式,使用 ${} 语法。
const name = "Alice";
const age = 30;
console.log(`My name is ${name} and I'm ${age} years old.`);
// 输出: My name is Alice and I'm 30 years old.
# 4. 表达式计算
插值表达式中可以包含任何有效的JavaScript表达式。
const a = 5;
const b = 10;
console.log(`The sum of ${a} and ${b} is ${a + b}.`);
// 输出: The sum of 5 and 10 is 15.
# 5. 调用函数
插值表达式中可以调用函数。
function capitalize(str) {
return str.charAt(0).toUpperCase() + str.slice(1);
}
const fruit = "apple";
console.log(`I love ${capitalize(fruit)}s.`);
// 输出: I love Apples.
# 6. 嵌套模板
模板字符串可以嵌套使用。
const nested = `This is ${`a nested ${`template`} string`}.`;
console.log(nested);
// 输出: This is a nested template string.
模板字符串提供了一种更简洁、更灵活的方式来处理字符串,特别是在需要插入变量或表达式的情况下。它们在现代JavaScript开发中被广泛使用,尤其是在构建动态内容和格式化输出时。
扫码关注(有广告,介意请勿关注)
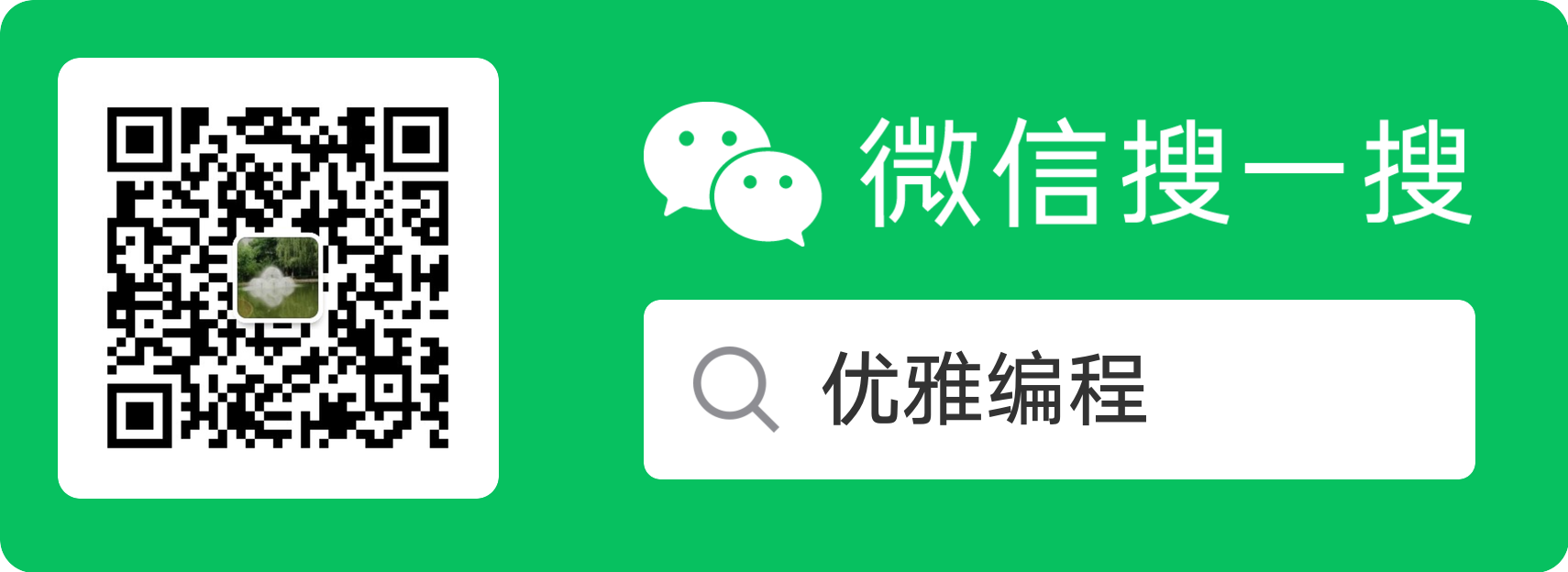
← Const/Let/Var 箭头函数 →