# 解构
解构赋值是 ES6 引入的一种语法,允许我们从数组或对象中提取值,并赋给变量。这种语法可以大大简化代码,提高可读性。
# 1. 数组的解构赋值
数组解构允许我们将数组的元素赋值给变量。
// 基本用法
const [a, b] = [1, 2];
console.log(a, b); // 输出: 1 2
// 忽略某些值
const [x, , y] = [1, 2, 3];
console.log(x, y); // 输出: 1 3
// 交换变量值
let m = 1, n = 2;
[m, n] = [n, m];
console.log(m, n); // 输出: 2 1
// 解构默认值
const [p = 5, q = 7] = [1];
console.log(p, q); // 输出: 1 7
# 2. 对象的解构赋值
对象解构允许我们将对象的属性赋值给变量。
// 基本用法
const {name, age} = {name: 'Alice', age: 30};
console.log(name, age); // 输出: Alice 30
// 给变量取别名
const {name: userName, age: userAge} = {name: 'Bob', age: 25};
console.log(userName, userAge); // 输出: Bob 25
// 解构默认值
const {city = 'Unknown', country = 'USA'} = {city: 'New York'};
console.log(city, country); // 输出: New York USA
# 3. 字符串的解构赋值
字符串可以被视为类数组对象,因此可以用数组或对象的方式解构。
// 按数组方式解构
const [a, b, c] = 'xyz';
console.log(a, b, c); // 输出: x y z
// 按对象方式解构
const {0: first, 1: second, length} = 'hello';
console.log(first, second, length); // 输出: h e 5
# 4. 函数参数的解构赋值
解构赋值也可以用于函数参数。
// 数组参数解构
function sum([a, b] = [0, 0]) {
return a + b;
}
console.log(sum([1, 2])); // 输出: 3
console.log(sum()); // 输出: 0
// 对象参数解构
function greet({name = 'Guest', greeting = 'Hello'} = {}) {
console.log(`${greeting}, ${name}!`);
}
greet({name: 'Alice', greeting: 'Hi'}); // 输出: Hi, Alice!
greet(); // 输出: Hello, Guest!
# 5. 解构赋值的注意事项
- 解构默认值仅在对应的值为 undefined 时生效。
- 如果解构模式中有变量名与属性名不一致,需要使用别名。
- 解构赋值可能会抛出错误,例如尝试解构 null 或 undefined。
- 默认值可以是表达式或者函数, 这种情况下表达式和函数是惰性的,优先使用结构的值
// 默认值示例
const [a = 1] = [undefined];
console.log(a); // 输出: 1
const [b = 1] = [null];
console.log(b); // 输出: null
// 错误示例
// const {prop} = null; // 这行会抛出 TypeError
const fun = () => {
console.log('fun')
return 2;
}
const [x = func()] = [1]
console.log(x); // 输出1
const [y = fun()] = []
console.log(y); // 输出2
通过合理使用解构赋值,我们可以编写更简洁、更易读的代码,特别是在处理复杂数据结构时。
扫码关注(有广告,介意请勿关注)
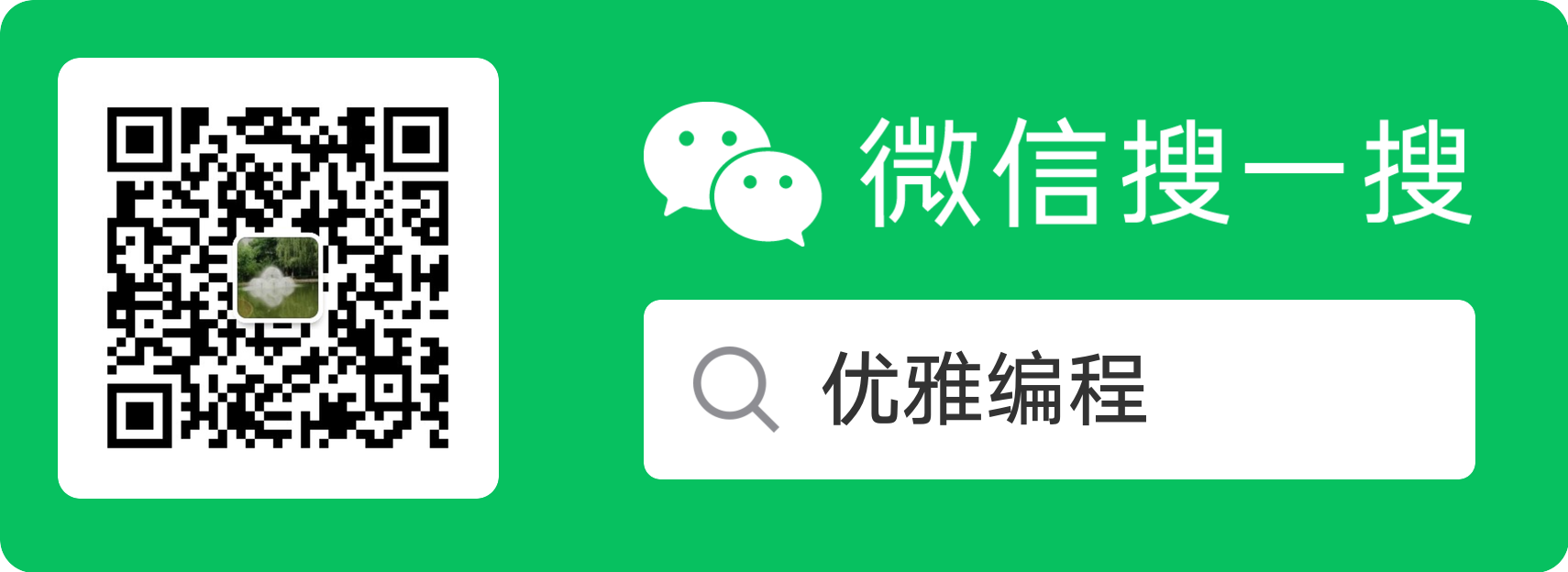